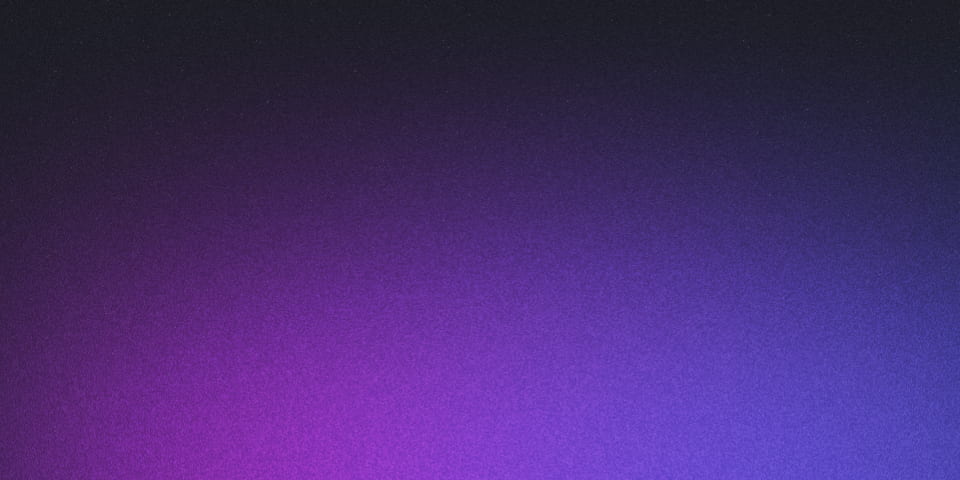
Building Pools: 101
Building a decentralized pool where users can deposit funds and receive a receipt token is a fundamental concept in DeFi. This pattern has been battle-tested in protocols like Uniswap, Aave, Compound, Balancer, and Curve. To do this efficiently, securely, and in a way that optimizes composability, we follow well-established best practices.
1. Architecture of the Pool
A well-structured pool should include the following components:
- Pool Smart Contract: Handles deposits, withdrawals, and tracking balances.
- Receipt Token (ERC-20 or ERC-4626): Represents a user’s share in the pool.
- Fee Mechanism (Optional): If applicable, include performance or withdrawal fees.
- Security Considerations: Implement reentrancy guards, access control, and gas optimizations.
- Interest or Yield Mechanism: If the pool is yield-bearing, it must integrate with strategies that generate returns for depositors.
- Accounting Model: Tracks users’ proportional ownership of assets, considering factors like variable deposits, withdrawals, and yield distribution.
2. Smart Contract Implementation Best Practices
a) Deposit Mechanism
Users deposit funds into the pool and receive receipt tokens proportional to their share.
function deposit(uint256 amount) external {
require(amount > 0, "Amount must be greater than 0");
IERC20(asset).transferFrom(msg.sender, address(this), amount);
uint256 shares = (amount * totalShares) / totalAssets;
_mint(msg.sender, shares);
totalAssets += amount;
}
b) Withdrawal Mechanism
Users burn their receipt tokens to redeem funds, ensuring withdrawals are proportional to their shares.
function withdraw(uint256 shares) external {
require(balanceOf(msg.sender) >= shares, "Insufficient shares");
uint256 amount = (shares * totalAssets) / totalShares;
_burn(msg.sender, shares);
IERC20(asset).transfer(msg.sender, amount);
totalAssets -= amount;
}
c) Yield-Bearing Strategies
If the pool generates yield, the protocol must fairly distribute earnings among depositors. A common approach is periodically updating share prices.
function distributeYield(uint256 yieldAmount) external onlyOwner {
require(yieldAmount > 0, "Yield must be greater than 0");
totalAssets += yieldAmount;
}
d) Using ERC-4626 (Tokenized Vault Standard)
Instead of reinventing the wheel, ERC-4626 provides an optimized vault standard for tokenized pools.
contract MyVault is ERC4626 {
constructor(IERC20 _asset) ERC4626(_asset) ERC20("My Pool Receipt Token", "MPRT") {}
}
Why ERC-4626?
- Automates share distribution
- Reduces gas costs for deposits/withdrawals
- Standardized for easier integrations
- Built-in accounting for yield-bearing pools
3. Security Best Practices
Security is non-negotiable when building financial primitives. Implement the following safeguards:
- Reentrancy Protection: Use
nonReentrant
from OpenZeppelin in functions handling deposits/withdrawals. - Access Control: Ensure only the contract or authorized accounts can mint/burn receipt tokens.
- Oracle Manipulation Prevention: If using price feeds, rely on Chainlink.
- Slippage Control: Implement min/max constraints for deposits and withdrawals.
- Upgradeable Contracts Considerations: Avoid vulnerabilities in proxy implementations.
- Use Rate Limits: Prevent sudden large-scale withdrawals that can disrupt the pool’s stability.
4. Governance and Fee Structures
For pools that involve governance or fee mechanisms, consider implementing:
- Governance Tokens: Users can vote on protocol changes.
- Performance Fees: A percentage of yield is allocated to the treasury or developers.
- Withdrawal Fees: To prevent excessive withdrawal churn.
function chargeWithdrawalFee(uint256 amount) internal returns (uint256) {
uint256 fee = (amount * withdrawalFeeBasisPoints) / 10000;
IERC20(asset).transfer(treasury, fee);
return amount - fee;
}
5. Battle-Tested Codebases to Study
- Uniswap V2/V3 – Liquidity pooling and LP tokens
- Aave aTokens – ERC-20 receipt tokens for lending pools
- Balancer Pool Contracts – Advanced liquidity pools
- OpenZeppelin ERC-4626 – Standardized vault implementation
- Yearn Vaults – Yield-bearing pool implementations
6. Deployment Considerations
- Use Foundry or Hardhat for development and testing.
- Run security simulations with Echidna or Slither.
- Audit contracts before deployment – Never skip this step.
- Consider Gas Optimization Techniques – Reduce redundant storage writes.
7. Composability and Integrations
A well-designed pool should integrate easily with other DeFi protocols:
- Compatible with Lending Protocols: Pools can serve as collateral for borrowing.
- LP Token Utility: Receipt tokens can be used in other DeFi strategies.
- Bridges and Cross-Chain Deployments: Consider L2 compatibility.
Conclusion
A well-designed liquidity pool ensures security, composability, and efficiency. Whether you’re building a lending protocol, a yield farm, or a simple staking contract, following these best practices will save you time and help you avoid costly mistakes. Make use of established standards like ERC-4626, integrate with existing DeFi primitives, and prioritize security at every step.